Difficulty |
3 |
Prerequisites |
Patterns are functions that associate a boolean value (typically interpreted as black and white) with every point.
bool pattern_function(const Point2D& point) // 2D
bool pattern_function(const Point3D& point) // 3D
We also know there exist operations on boolean values:
-
Conjunction
b1 && b2
. -
Disjunction
b1 || b2
. -
Negation
!b
.
In this extension, we find out what happens if we apply those logic operators on patterns.
Say we have two patterns p1
and p2
.
p1 | p2 |
---|---|
![]() |
![]() |
If we combine them using disjunction, we get
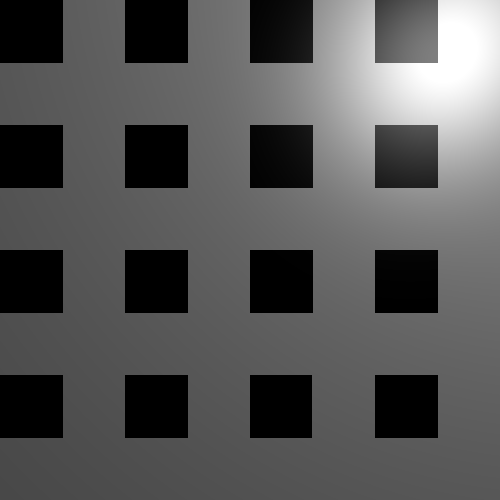
Notice how in the resulting image, a pixel is white if either one of the images above has a white pixel in the same spot.
1. Implementation
Create files |
1.1. 2D
1.1.1. Conjunction
Write a function
It implements the following table:
Make use of |
1.1.2. Disjunction
Write a function
It implements the following table:
Make use of |
1.1.3. Exclusive Disjunction
Write a function
It implements the following table:
Make use of |
1.1.4. Negation
Write a function
It implements the following table:
Make use of |
1.2. 3D
Implement the same logical operations, but now on 3D patterns.
Make use of |
2. Finishing Touches
Expose all these operations to the scripting language. |
3. Evaluation
Render the following scene: ![]()
|
-
Show that your implementations make use of the lambda pattern.