Difficulty |
3 |
Prerequisites |
|
As mentioned before, a pattern can be seen as a black and white image. But not just any black and white image, but rather an infinitely large one. For any position \((x, y)\) or \((x, y, z)\), the pattern must decide whether that spot is black or white.
In order to fill up this infinite space, we generally rely on repetition: one simple patterns gets copied an infinite number of times.
In this extension, you will have to create the "for loop" of patterns: the tiling
operation takes a "child pattern", a width and a height and will then copy this ad infinitum.
example
|
We start off we with simple pattern: ![]() Tiling will lead to this: ![]() |
1. Implementation
Create files Also add the header file to |
1.1. Tiling in 2D
Given a point \((x, y)\), you need to "remap" it to \([0,0] \times [w, h]\). This remapped point can then be fed to the child pattern.
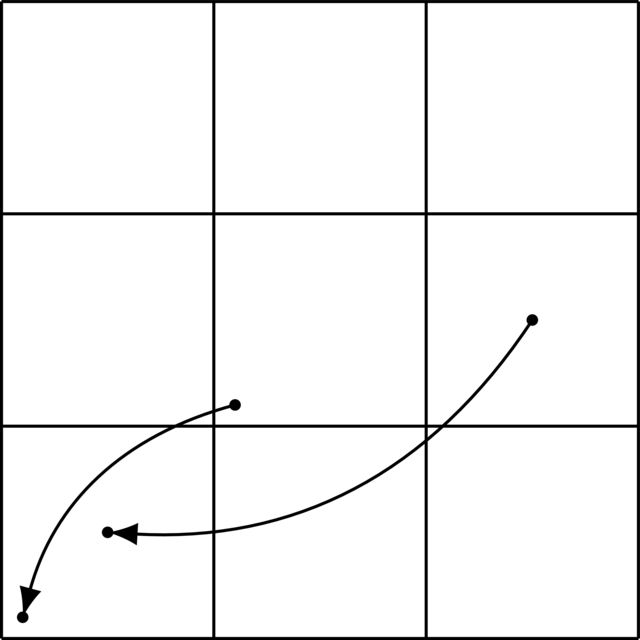
Implement tiling as a function
Rely on
|
Important
|
You might have some trouble to get the area around \((0,0)\) right. Say you are copying this pattern: ![]()
|
1.2. tiling_x, tiling_y
tiling
takes a rectangular pattern and copies it both horizontally and vertically.
What if we already have an infinitely wide pattern and only need to copy it vertically, or vice versa?
Implement horizontal and vertical tiling as two functions
Rely on |
1.3. Tiling in 3D
The same trick can be applied in 3D. We get a "pattern box" which is copied along all three axes.
Implement 3D tiling as
Rely on |
1.4. Finishing Touches
Add bindings to expose this new functionality to the scripting language. |
2. Evaluation
Use
![]() ![]()
|
Show that your code for |