Difficulty |
2 |
Reading material |
Important
|
Making this extension only makes sense if you also make the Edge Renderer (which in itself leads to the Masking Renderer). |
1. Goal
The purpose of the Grouper
primitive can better understood in the context of Masking Renderer.
Here’s a video:
As you can see, there are black contours to the image. In this case, we can simply add a contour around every primitive.
However, sometimes a single "entity" is made out of multiple primitives, such as a mesh. If we were to draw a contour around every primitive, every triangle in the mesh would have one.
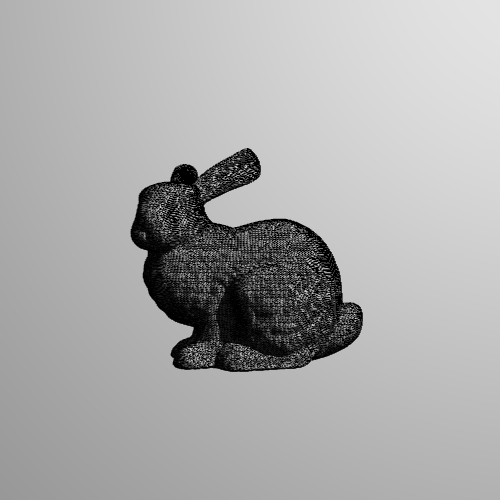
Instead, we want to see the bunny as a whole and have only one contour:
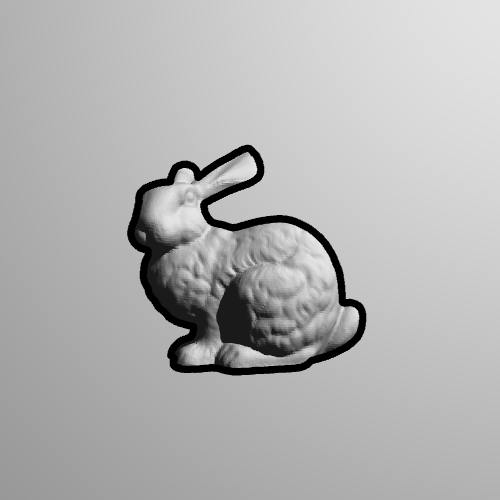
2. Grouper Primitive
In order to tell the ray tracer which primitives form a whole, we need to put them in the same group. For example, to produce the image of the first bunny (with contours around every triangle), each triangle resided in its own separate group, whereas the second bunny had all triangles placed in the same group.
For this extension, it is not important how to draw the contour. We are only interested in defining groups.
Go find the code of |
2.1. Skeleton
Create files
|
2.2. bounding_box
Let’s start with the easiest part: bounding_box
.
Its implementation should be obvious.
Implement |
2.3. find_all_hits
find_all_hits
should ask the child primitive for all hits and overwrite all group_id
fields with the Grouper
's id.
Implement |
3. Evaluation
There are two ways to implement
What difference does this cause? Can you relate this to CSS? |
Write tests that check the following:
|