Difficulty |
2 |
Reading material |
|
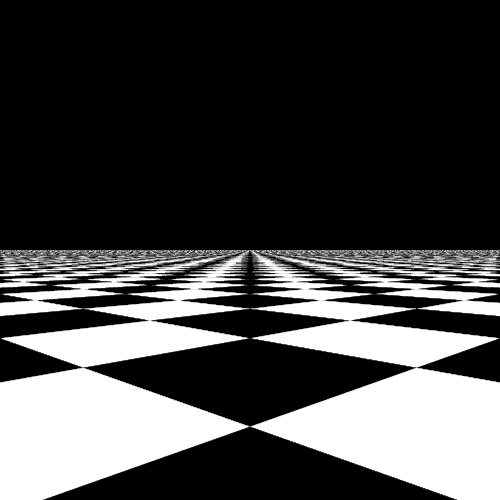
var camera = Cameras.perspective( [ "eye": pos(5, 0.5, 5),
"look_at": pos(0, 0.5, 0),
"up": vec(0, 1, 0),
"distance": 1,
"aspect_ratio": 1 ] )
var white_material = Materials.uniform( [ "ambient": Colors.white(),
"diffuse": Colors.black(),
"specular": Colors.black(),
"specular_exponent": 0 ] )
var black_material = Materials.uniform( [ "ambient": Colors.black(),
"diffuse": Colors.black(),
"specular": Colors.black(),
"specular_exponent": 0 ] )
var checkered_material = Materials.from_pattern(Patterns.checkered(1, 1), white_material, black_material)
var root = decorate(checkered_material, xz_plane())
var lights = [ Lights.omnidirectional( pos(0, 10, 0), Colors.white() ) ]
var scene = create_scene(camera, root, lights)
var raytracer = Raytracers.v6()
var renderer = Renderers.standard( [ "width": 500,
"height": 500,
"sampler": Samplers.random(8),
"ray_tracer": raytracer ] )
pipeline( scene,
[ Pipeline.renderer(renderer),
Pipeline.studio() ] )
1. Goal
The purpose of samplers is explained on the page discussing ray tracing concepts.
In short, we have a pixel for which we need to determine its color. We have to cast a ray through it which
we’ll trace around the scene. But since the pixel is not a single point but is rectangular, there are infinitely
many points through which we could cast the ray.
A sampler’s job is to pick points within the pixel. The number of points and which points these are is up to the sampler.
The only guarantee the sampler has to give is that when given a 2D rectangle, it will produce points
that are within this rectangle. Go take a look at samplers/sampler.h
. The (first) sample
method reflects
this purpose: it takes a rectangle and returns a list of points. You can ignore the second sample
overload for now.
The random sampler can be parameterized with an integer n
.
When asked to sample a rectangle, it will return n
random points in this rectangle.
Create new files
|
Expose the sampler to the scripting language by updating |
2. Evaluation
Render the following scene:
Explain why there is such noise on the circumference of the circle. |
Render the following scene:
Explain the change in noise. What would you do to get rid of the noise altogether? |