Difficulty |
3 |
Prerequisites |
|
Reading material |
|
1. Introduction
Numbers have operations defined on them: we can add two numbers together (\(a + b\)), subtract them (\(a - b\)), multiply them (\(a \times b\)), and so on. Similary, we can apply operations on primitives: take their union, their difference, their intersection, we can translate, scale and rotate them, and so on. The same can be done with animations.
In this extension, you are tasked with defines a series of operations that act upon animations.
2. Limit
Sometimes, we just want to cut off an animation prematurely. Say we have an animation of duration 10:
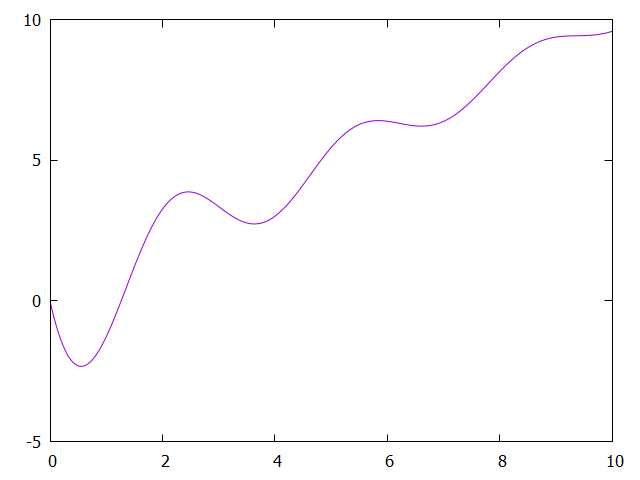
We can choose to cut it off after 5 seconds:
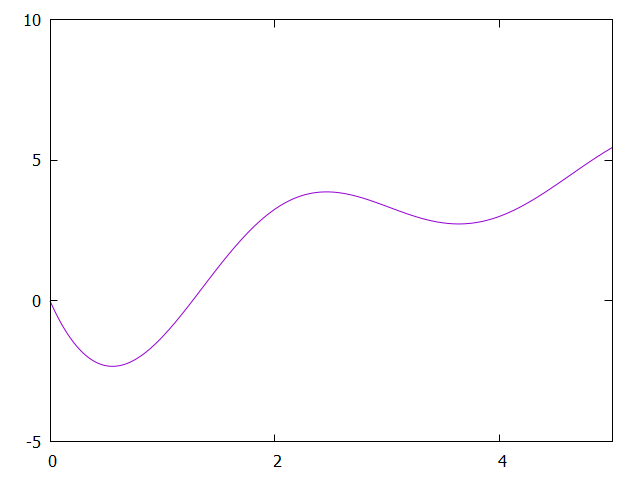
Create a file
|
Tip
|
First examine |
3. Invert
An animation can be inverted: instead of going from A to B, it goes from B to A.
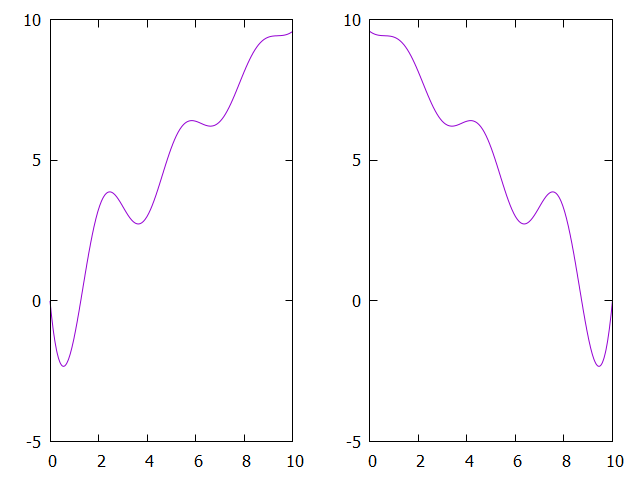
Create a file
that returns the inversion of the given animation |
4. Sequence
Animations can be put in sequence. For example, consider the two animations below, each lasting 10 seconds:
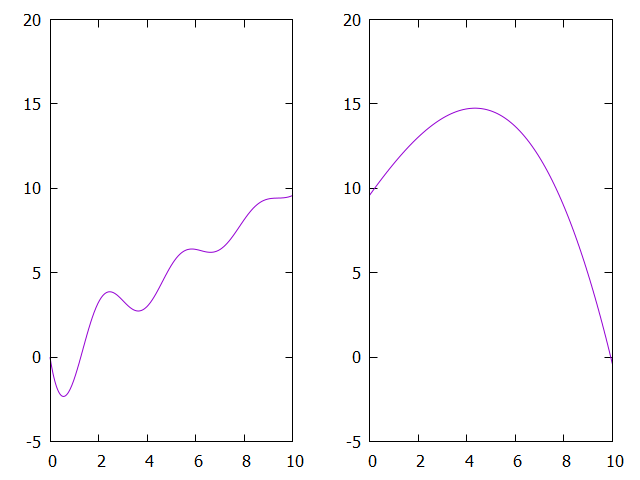
Sequencing them yields
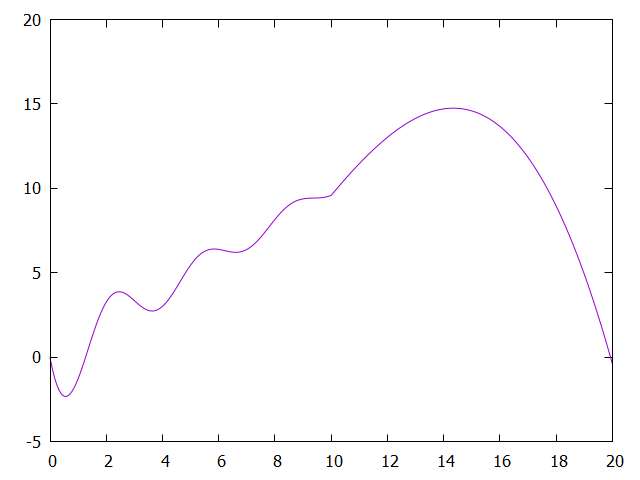
Typically, you will only combine animations if they have matching endpoints (ending point of the first is equal to the starting point of the second), but this is not mandatory.
Create a file
|
5. Looper
An animation can be repeated at infinitum. For example, take the animation below:
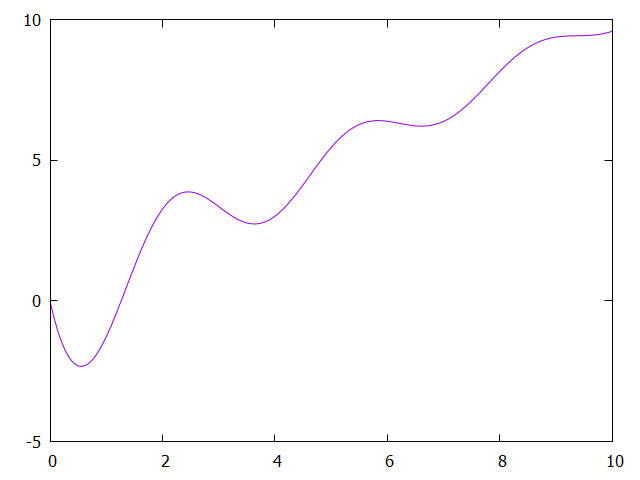
Repeating this animation endlessly gives a new animation with infinite duration:
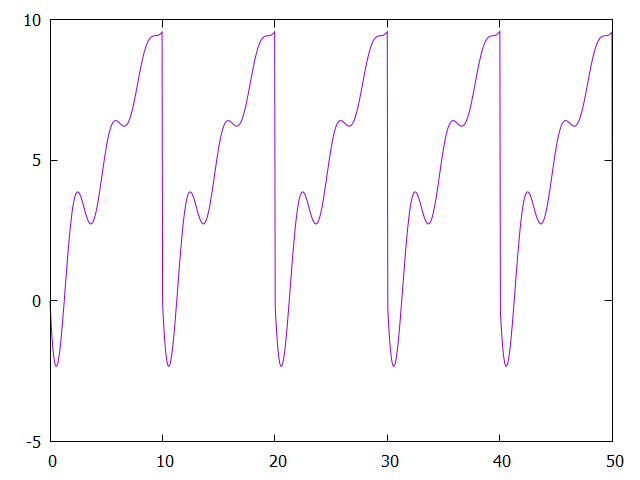
Create a file
|
6. Cycle
Looping an animation is nicer if the starting and ending point coincide, otherwise a sudden jump will occur when the animation resets. One way to easily achieve this is to sequence an animation with its inversion.
Consider the animation below:
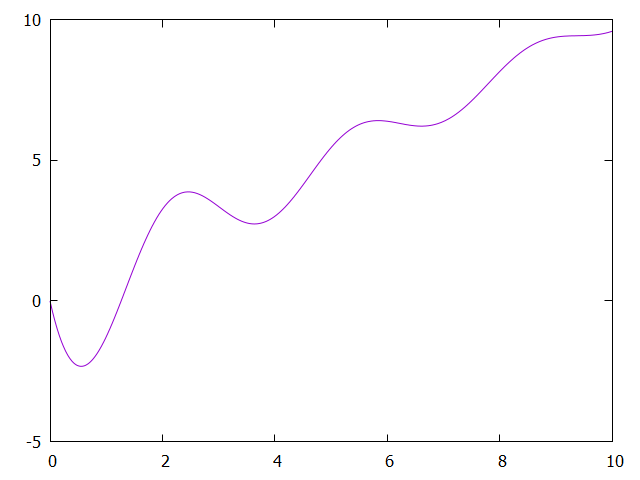
We take its inverse:
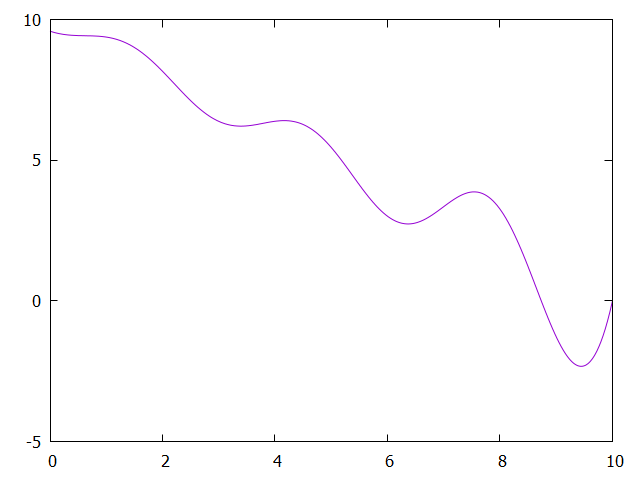
We sequence them:
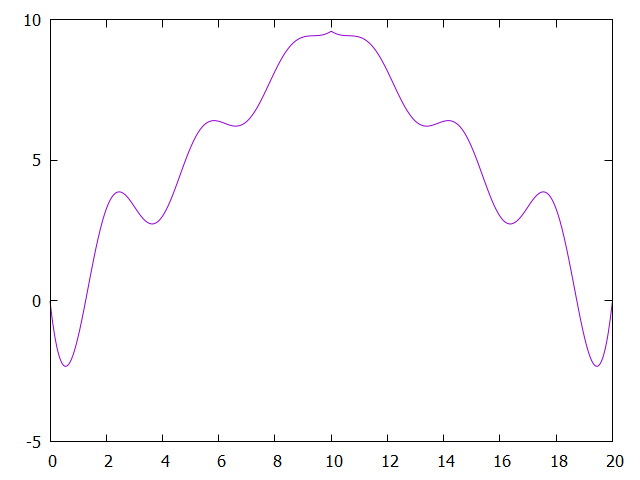
Finally, we loop it:
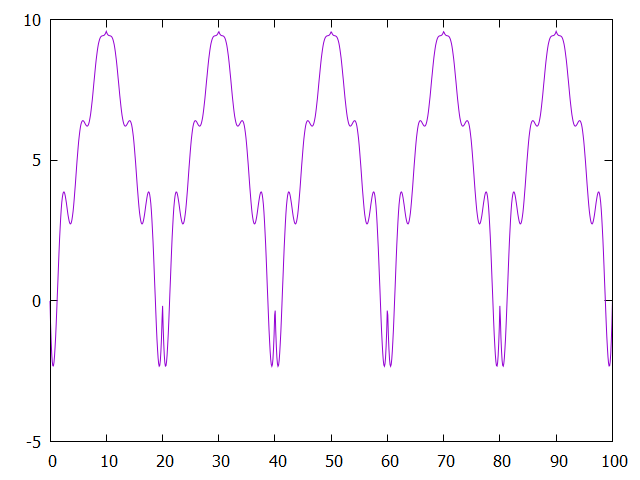
Create a file
|
7. Bindings
Expose each of these functions to the scripting language.
Since we support animations of different types ( For example, say you want to export
Next, you will need to add a binding for all supported types:
|
8. Evaluation
Reproduce the following animation: |
Reproduce the following animation: |
Reproduce the following animation: |
Render the scene below.
|